Programmingempire
This article demonstrates several Examples of For-each Loop in Java. In fact, the use of for-each loop makes traversing the elements of a collection much simplified. The following code shows a simple example of displaying the elements of an array using the for-each loop.
int[] arr= {5, 9, 10, 1, 6, 7, 3};
System.out.println("Array Elements...");
for(int k: arr)
{
System.out.print(k+" ");
}
As can be seen, there is no loop control variable to track the array index. In fact, it is not needed in a for-each loop. Given that, we specify a variable of the same type as the type of array elements, it is assigned the successive array element in each iteration. In this example, the variable k will get the value of each array element in the successive iteration. Moreover, there is no need for condition checking to determine when all elements are traversed. The for-each loop automatically performs this condition checking and terminates when all elements are visited.
Syntax of For-each Loop in Java
Basically, the loop starts with the for keyword. Also, it is required to specify the data type followed by the name of the loop control variable in the paranthesis. Further, the name of the collection should be specified followed by a : symbol as shown below.
for(data-type variable-name : collection-name)
{
//statements
}
However, there are certain important points regarding for-each loop that must be remembered. Firstly, you can not modify any element of the given collection. Because elements of the collection are read-only within the for-each loop. Secondly, the loop will terminate by itself when all elements are visited. However, it is possible to terminate the loop in-between using the break statement.
Another example of using for-each loop in Java is given below. As can be seen, in the example, we are using the for-each loop to traverse an ArrayList. Furthermore, the ArrayList is a generic collection that takes a specific data type as a parameter. So, we have specified Object as the type of its elements. Therefore, now we can add elements of any type to the list. The following code demonstrates it.
//Creating an ArrayList
ArrayList<Object> mylist=new ArrayList<Object>(); mylist.add("ABC");
mylist.add(200);
mylist.add("BCA");
mylist.add(1.25259);
mylist.add('x');
mylist.add(true);
mylist.add(10);
for(Object o: mylist)
{
System.out.print(o+" ");
}
As an illustration, the following code shows traversing the elements of a LinkedList using for-each loop. Also, the LinkedList is generic and takes String as the type of parameter.
//Creating Linked List
LinkedList<String> IndianCities=new LinkedList<String>();
IndianCities.add("Delhi");
IndianCities.add("Mumbai");
IndianCities.add("Bangalore");
IndianCities.add("Jammu");
IndianCities.add("Kolkata");
IndianCities.add("Dispur");
IndianCities.add("Chennai");
for(String s: IndianCities)
{
System.out.print(s+" ");
}
The Program Illustrating For-each Loop in Java
The following code shows the complete example.
package CollectionExamples;
import java.util.*;
public class MyCollections {
public static void main(String[] args) {
int[] arr= {5, 9, 10, 1, 6, 7, 3};
System.out.println("Accessing Array Elements...");
for(int k: arr)
{
System.out.print(k+" ");
}
System.out.println();
//Creating an ArrayList
ArrayList<Object> mylist=new ArrayList<Object>();
mylist.add("ABC");
mylist.add(200);
mylist.add("BCA");
mylist.add(1.25259);
mylist.add('x');
mylist.add(true);
mylist.add(10);
System.out.println("Accessing ArrayList Elements...");
for(Object o: mylist)
{
System.out.print(o+" ");
}
System.out.println();
//Creating Linked List
LinkedList<String> IndianCities=new LinkedList<String>();
IndianCities.add("Delhi");
IndianCities.add("Mumbai");
IndianCities.add("Bangalore");
IndianCities.add("Jammu");
IndianCities.add("Kolkata");
IndianCities.add("Dispur");
IndianCities.add("Chennai");
System.out.println("Accessing LinkedList Elements...");
for(String s: IndianCities)
{
System.out.print(s+" ");
}
System.out.println();
}
}
Output

- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
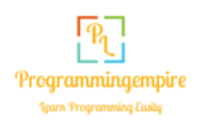