Programmingempire
In this post on How to Create and Use Arrow Functions in TypeScript, I will explain the concept of arrow functions in TypeScript. Basically, arrow functions represent the functions which don’t have a name. In other words, arrow functions are anonymous functions.
Why Do We Need Arrow Functions?
Although we create functions for the purpose of reusability of the code, still there are certain situations where we don’t require to use a function more than once. For instance, suppose we need to define a function at someplace in the code and subsequently invoke that function. Apart from that use, we are not going to use that function again in our application. In such a case, defining an anonymous function is more suitable. With this purpose in mind, we can create an arrow function that gives us a concise syntax to create small functions.
Syntax for Defining Arrow Functions in TypeScript
The TypeScript uses following syntax for creating an arrow function. Also, we can have arrow functions having a single expression or single statement as well as multiple statements. Example of an arrow function having a single expression is given below.
//Single Statement Arrow Function
let variable_name=(parameter_list)=>expression;
//Example
let v=(i:number)=>i*i;
As said earlier, an arrow function may comprise of several statements as shown below.
let v=()=>{
let x:number=5;
let y: number=12;
let z:number;
z=x+y;
console.log(z);
}
Examples of Arrow Functions
In fact, we can use arrow functions both within the class as well as outside the class as an ordinary function. Moreover, it is possible to create an arrow function with parameters as well as without parameters.
The following code shows several examples of creating and calling arrow functions. To begin with this example, we create an arrow function with no parameter and also no returning value. It just displays the string “Hello, World!”. After that, we create another arrow function that increments the parameter and returns it. The third arrow function finds the square root of its parameter, while the fourth one finds power. The next arrow function returns the factorial of its parameter, Finally, the last arrow function returns the sum of all integers from 1 to the given number.
let v1=()=>console.log("Hello, World!");
console.log("Display a String: ");
v1();
let v2=(x:number):number=>++x;
console.log("Increment a Number: ");
console.log(v2(9));
let v3=(x:number):number=>Math.sqrt(x);
console.log("Display Square Root of 10: ");
console.log(v3(10));
let v4=(x:number, y:number)=>Math.pow(x, y);
console.log("Display 1.9 raise to the power 2: ");
console.log(v4(1.9, 2));
let v5=(x:number)=>{
let f:number=1;
for(let i=1;i<=x;i++)
f=f*i;
return f;
}
console.log("Display Factorial of 7: ");
console.log(v5(7));
let v6=(x:number)=>{
let s=0;
for(let i=1;i<=x;i++)
s+=i;
return s;
}
console.log("Display sum of all integers from 1 to 12: ");
console.log(v6(12));
Output

An example of using Arrow functions in a class is shown below. As an illustration, the class Student contains several properties. Also, it has a constructor and three functions. However, the three functions defined in the class are created as arrow functions that we explain next.
Firstly, the function info() returns a string containing information about the Student object. Secondly, the function findtotal() returns the total marks of a student. Finally, the findpercentage() function returns the percentage.
class Student
{
studentid: number;
sname: string;
marks1: number;
marks2: number;
marks3: number;
total_marks:number;
percentage:number;
course: string;
constructor(id:number, sn:string, m1:number, m2:number, m3:number, cr:string)
{
this.studentid=id;
this.sname=sn;
this.marks1=m1;
this.marks2=m2;
this.marks3=m3;
this.course=cr;
}
info=()=>{
let str:string;
str="Student ID: "+this.studentid+", Student Name: "+this.sname+", Course:"+this.course;
return str;
}
findtotal=()=>this.marks1+this.marks2+this.marks3;
findpercentage=()=>{
this.total_marks=this.marks1+this.marks2+this.marks3;;
return this.total_marks/3.0;
}
}
var ob=new Student(10, "Aman", 75, 89, 58, "BBA");
console.log("Student Information: ");
console.log(ob.info());
console.log("Total Marks: ");
console.log(ob.findtotal());
console.log("Percentage: ");
console.log(ob.findpercentage()+"%");

Summary
In this article on How to Create and Use Arrow Functions in TypeScript, I have explained the concept of arrow functions, their benefits, and usage. Additionally, the arrow functions are explained by the demonstration of several examples of creating them in several different ways.
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
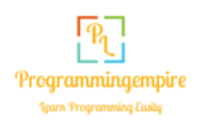