Programmingempire
In this post on Introducing Decorators in TypeScript, I will give an introduction to the concept of decorators in TypeScript. In fact, this introductory article contains information about the decorator concept, its various types, and the benefits of using decorators. If you are using TypeScript version 1.5 or later, then you can use this feature. Furthermore, you can know the version of TypeScript you are working with by using the following command.
tsc -v
For instance, you can get an output like the following.

What is a Decorator?
Basically, a decorator is a construct that is used to decorate classes and functions. In fact, a decorator is just a function and we use it when we need to customize our class at design time. Additionally. decorators attach the meta-data to the entities they are associated with.
Why do we need Decorators?
Indeed, decorators offer a metaprogramming syntax. Therefore, we first need to understand what is meant by metaprogramming?
Metaprogramming
Suppose you want to modify a construct of the current program such as a class or a function while the program is still running, then you can use the metaprogramming technique. By using metaprogramming, you get the desired flexibility, however, your code becomes difficult to understand. Also, for the casual programmer, it is of little use.
In TypeScript, we have available with us the Decorator syntax for supporting the metaprogramming. Additionally, decorators provide annotations for the classes as well as to the members of the classes. In essence, the decorators indicate a function for a specific construct in TypeScript.
Categories of Decorators in TypeScript
In general, we can have decorators for different programming constructs. Accordingly, we can categorize decorators as follows.
- Class Decorators
- Method Decorators
- Property Decorators
- Parameter Decorators
- Accessor Decorators
Now that, we have an understanding of decorators, let us consider each decorator one by one. With this purpose in mind, we begin with the Class Decorators.
Basically, a class decorator refers to a function that will be called at runtime with the constructor of the class. Hence, whenever we create an object of the class, and its constructor is executed, then the corresponding function associated with the class decorator also gets executed. Also, the class decorator function is executed before any other statement in the constructor gets executed.
In the same way, we can attach a decorator with a function that associates the corresponding annotation with that function.
Similarly, if we need to associate some metadata with a property, we can use Property Decorator.
Likewise, we also have the provision of associating metadata with method parameters in the form of Parameter Decorators that we also create using a function.
Lastly, we can have accessor decorators that we can associate with both get and set accessors for a class member. As a matter of fact, these decorators also add annotations to the accessors.
Example of Using a Class Decorator
Now let us consider a simple example of a Class Decorator. In the following example, we create a class called MyClass and associate a decorator with the name a to this class. Further, create a class decorator function with the same name a. Now, save the program and run it without creating any object of the class.
function a(m:string): ClassDecorator{
console.log(`A String in Decorator: ${m}`);
return function():void{
console.log('A Modification in Constructor');
}
}
@a('Argument to the Decorator')
class MyClass
{
x:number;
y:number;
constructor(x:number, y:number)
{
console.log("Inside the constructor");
this.x=x;
this.y=y;
console.log(`The sum of ${this.x} and ${this.y} is ${this.x+this.y}`);
}
}
//let ob=new MyClass(12, 56);
Output

As you can see in the output, we don’t create any object of the class. Still, the Class Decorator function gets executed. Now, remove the comment from the last statement and compile and run the program again as shown below.
let ob=new MyClass(12, 56);
As can be seen from the output shown below, now the statements in the constructor also get executed along with the statements in the decorator function.
Output

Summary
In this article on Introducing Decorators in TypeScript, I have explained the concept of metaprogramming and decorators in TypeScript. Also, I discussed different types of decorators and their significance. In essence, the decorators are nothing but the functions that add some metadata to a construct. In the next article, I will demonstrate the working of decorators using different examples.
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
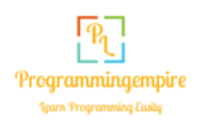