Programmingempire
In this post on Learning Property Decorator in TypeScript with Examples, I will explain property decorators with a few examples. Earlier, I have explained the concept of decorators in TypeScript and also described the Class and Method decorators. In the same way, we can use decorators with the properties of the class.
Now, first, let us understand how to add a Property Decorator to a class property. Given these points, first, we create a class called Student as given below.
class Student
{
student_id: number;
student_name: string;
constructor(sid:number, sname: string)
{
this.student_id=sid;
this.student_name=sname;
}
}
After that, add a property decorator called prop to one of the properties of the class. This time, we add the decorator to the Student_id property as shown below.
@prop
student_id: number;
In addition, we define the corresponding decorator function as follows.
function prop(target:Object, propertyKey:string): any
{
console.log('Inside Property Decorator Function');
}
Now, the complete code looks as follows. Meanwhile, save the code as .ts file and compile it. Once, code is compiled, run it to get the following output.
function prop(target:Object, propertyKey:string): any
{
console.log('Inside Property Decorator Function');
}
class Student
{
@prop
student_id: number;
student_name: string;
constructor(sid:number, sname: string)
{
this.student_id=sid;
this.student_name=sname;
}
}
Output

Further, we add the necessary functionality in our decorator function. For this purpose, we need to define the get and set accessors of the property. Hence, we update the function prop as follows.
function prop()
{
return function(target:Object, propertyKey: string){
console.log('Inside Property Decorator Function');
let value: number;
Object.defineProperty(target, propertyKey, {
get: () =>{
console.log("Inside get accessor");
return value;},
set: (v: number) => {
console.log("Inside set accessor");
value=v;}
});
}
}
It is important to realize that the property decorator function takes two arguments. While the first one with the name as target represents either the constructor function of the class or the prototype of the class given that the class member is whether a static member or an instance member respectively.
On the other hand, the second argument with the name propertyKey represents the name of the property being decorated. Afterward, we call the Object.defineProperty() method in order to create the new property definition.
Object.defineProperty()
Basically, we use this method in our Property Decorator function. As a matter of fact, it defines a new property or makes changes in an existing one on a specific object. As mentioned in the above code, this method takes three parameters. The first one represents a particular object on which the property is defined. While the second one represents the property name and the last one indicates the property descriptor containing the get and set accessors. Now that, we can complete our code which is given below.
function prop()
{
return function(target:Object, propertyKey: string){
console.log('Inside Property Decorator Function');
let value: number;
Object.defineProperty(target, propertyKey, {
get: () =>{
console.log("Inside get accessor");
return value;},
set: (v: number) => {
console.log("Inside set accessor");
value=v;}
});
}
}
class Student
{
@prop()
student_id: number;
student_name: string;
constructor(sid:number, sname: string)
{
this.student_id=sid;
this.student_name=sname;
}
}
let ob=new Student(1, "ABC");
console.log(ob.student_id);
console.log(ob);
ob.student_id=2;
console.log(ob.student_id);
console.log(ob);
Output

To elaborate this concept further, let us consider another example. In like manner, we create a class and populate it with some properties and the constructor function. However, this time we create the decorators for two properties as shown in following code.
function d1(){
return function(target:Object, propertyKey: string)
{
console.log('Inside Property Decorator for x');
let v: number;
const MyGetter = function(){
return v;
};
const MySetter = function(n: number){
if((n<5) || (n>15)){
Object.defineProperty(target, 'xerrors', {value: `The value should be in the range [5-15]` });
}
else{ v = n; }
};
Object.defineProperty(target, propertyKey, {
get: MyGetter,
set: MySetter
});
}
}
function d2(m: number){
return function(target:Object, propertyKey: string)
{
console.log('Inside Property Decorator for y');
let value: number;
const MyGetter1 = function(){
return this[propertyKey + 'value'];
};
const MySetter1 = function(n: number){
if(n!=m){
Object.defineProperty(target, 'yerrors', {value: `The value should be Equal to 100` });
}
else{ this[propertyKey + 'value'] = n;}
};
Object.defineProperty(target, propertyKey, {
get: MyGetter1,
set: MySetter1,
enumerable: true,
configurable: true
});
}
}
class MyClass
{
@d1()
x: number;
@d2(100)
y: number;
constructor(x:number, y:number){
this.x=x;
this.y=y;
console.log(`x = ${this.x}, y = ${this.y}`);
}
}
console.log("First Object: ");
let ob=new MyClass(2, 125);
console.log(ob.xerrors);
console.log(ob.yerrors);
console.log();
console.log("Second Object: ");
let ob1=new MyClass(80, 100);
console.log(ob1.xerrors);
console.log(ob1.yerrors);
console.log();
console.log("Third Object: ");
let ob2=new MyClass(12, 100);
console.log(ob2.xerrors);
console.log(ob2.yerrors);
console.log();
console.log("Fourth Object: ");
var ob3=null;
ob3=new MyClass(14, 90);
console.log(ob3.xerrors);
console.log(ob3.yerrors);
In short, we can list important points about the above code.
- Firstly, we create property decorators for both the properties x and y with names d1 and d2 respectively.
- In particular, d2 passes the parameter with the value 100. However, d1 doesn’t have any argument.
- Also, the set accessors for both properties use a condition to check whether some valid value is being assigned to the corresponding properties or not.
- Lastly, the assignment of a value in set accessor of d2 takes care that the value corresponding to the current context is being assigned.
After that, we create few objects of the class and display the values of newly created properties xerrors, and yerrors.
Output

Summary
In this article on Learning Property Decorators in TypeScript with Examples, I have explained the concept and implementation of Property Decorators in TypeScript by citing a few examples. I hope, it will help you in creating some property decorators of your own.
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
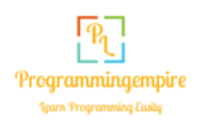