Programmingempire
This article describes Methods of Array Class in C#. Basically, the Array class is contained in the library of the .NET framework and provides functionalities for supporting arrays in C#. In general, the Array class contains methods to create arrays. Moreover, it also provides methods to perform certain operations on arrays.
Creating an Array
In order to create an array using the Array class, we can use its static method CreateInstance(). Basically, this method takes the type of array, and its length as parameters and returns an instance of the corresponding array. However, this method has five more overloads. The following example shows the use of the CreateInstance() method.
Array arr1 = Array.CreateInstance(typeof(System.Int32), 6);
Likewise, we can create a 2D array using another overload of the CreateInstance() method as follows. Here we need to specify the size of both dimensions.
Array m1 = Array.CreateInstance(typeof(System.Int32), 2, 3);
Assigning Values to an Array and Retrieving Values
In order to assign the value to an array element, we can use the SetValue() method. This method takes the value to be assigned and the corresponding index as its two parameters. The following example shows the use of this method.
arr1.SetValue(23, 0);
Similarly, we can assign a value to an element of a 2D array as follows. Basically, it assigns the value 23 to the 0th element.
m1.SetValue(i + j, i, j);
where i, and j represent the index of the array element. Similarly, we can retrieve an element of an array by using the GetValue() method. The following code shows an example of a 1D as well as a 2D array.
//Retrieving value from a 1D array
arr1.GetValue(i)
//Retrieving value from a 2D array
m1.GetValue(i, j)
// here i, and j represent the index of the element being accessed
Sorting an Array
Further, the Array class contains the Sort() method to sort the elements of the array like the following example shows.
//Sorting Array
Array.Sort(arr1);
Reversing an Array
Similarly, we can reverse the elements of the array like the following example shows.
//Reversing Array
Array.Reverse(arr1);
The Complete Program to Demonstrate Methods of Array Class
using System;
namespace ArrayClassDemo
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Creating an object of the Array class...");
Array arr1 = Array.CreateInstance(typeof(System.Int32), 6);
arr1.SetValue(23, 0);
arr1.SetValue(67, 1);
arr1.SetValue(47, 2);
arr1.SetValue(98, 3);
arr1.SetValue(239, 4);
arr1.SetValue(57, 5);
Console.WriteLine("1D Array...");
for(int i=0;i<arr1.Length;i++)
{
Console.Write(arr1.GetValue(i)+" ");
}
//Sorting Array
Array.Sort(arr1);
Console.WriteLine("\nSorted 1D Array...");
for (int i = 0; i < arr1.Length; i++)
{
Console.Write(arr1.GetValue(i)+" ");
}
//Reversing Array
Array.Reverse(arr1);
Console.WriteLine("\nReversed 1D Array...");
for (int i = 0; i < arr1.Length; i++)
{
Console.Write(arr1.GetValue(i) + " ");
}
// Creating a 2D array
Array m1 = Array.CreateInstance(typeof(System.Int32), 2, 3);
for(int i=0;i<2;i++)
{
for (int j = 0; j<3;j++)
{
m1.SetValue(i + j, i, j);
}
}
Console.WriteLine("\n2D Array...");
for(int i = 0; i <= m1.GetUpperBound(0); i++)
{
for(int j=0;j<=m1.GetUpperBound(1);j++)
{
Console.Write(m1.GetValue(i, j) + " ");
}
Console.WriteLine();
}
}
}
}
Output

Further Reading
How to Create Instance Variables and Class Variables in Python
Comparing Rows of Two Tables with ADO.NET
Example of Label and Textbox Control in ASP.NET
One Dimensional and Two Dimensuonal Indexers in C#
Private and Static Constructors in C#
Programs to Find Armstrong Numbers in C#
One Dimensional and Two Dimensional Indexers in C#
Generic IList Interface and its Implementation in C#
Creating Navigation Window Application Using WPF in C#
Find Intersection Using Arrays
An array of Objects and Object Initializer
Performing Set Operations in LINQ
Data Binding Using BulletedList Control
Understanding the Quantifiers in LINQ
Deferred Query Execution and Immediate Query Execution in LINQ
Examples of Query Operations using LINQ in C#
An array of Objects and Object Initializer
Language-Integrated Query (LINQ) in C#
Examples of Connected and Disconnected Approach in ADO.NET
IEnumerable and IEnumerator Interfaces
KeyValuePair and its Applications
Learning All Class Members in C#
Examples of Extension Methods in C#
How to Setup a Connection with SQL Server Database in Visual Studio
Understanding the Concept of Nested Classes in C#
A Beginner’s Tutorial on WPF in C#
Explaining C# Records with Examples
Everything about Tuples in C# and When to Use?
Linear Search and Binary search in C#
Examples of Static Constructors in C#
When should We Use Private Constructors?
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
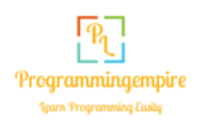