Programmingempire
In this post, I will discuss several New Features of C# 9. In fact, the latest release of C# has added many new features for making coding easier. Here, I will explain Records, Init-Only properties, Top-Level statements, module initializers, and use of new().
Following are the New Features in C# 9
The important New Features in C# 9 are discussed below.
Records
Basically, records are immutable reference types. The objects of the records are compared on the basis of their content rather than reference. As an illustration, an example of creating records is given below.
using System;
namespace ConsoleApp42
{
record MyRecord
{
public int x { get; init; }
}
class Program
{
static void Main(string[] args)
{
MyRecord ob1 = new MyRecord { x = 20 };
MyRecord ob2 = new MyRecord { x = 20 };
Console.WriteLine((ob1 == ob2));
Console.WriteLine((ob1 != ob2));
}
}
}
Output

As can be seen, the two records are compared for equality on the basis of the values stored in the property x rather than their references.
Init-Only Properties
Generally, we use object initializers to avoid creating constructors. However, the properties need to be readable and writable for creating objects. However, with C# 9.0, we don’t need to have set accessors. Instead, we can use an init accessor with properties so that we can create objects using object-initializers. After that, the properties become immutable as shown in the following example.
using System;
namespace ConsoleApp39
{
class A
{
public int X { get; init; }
public int Y { get; init; }
public override string ToString()
{
return $"Sum ={X+Y}";
}
}
class Program
{
static void Main(string[] args)
{
A ob = new A { X = 55, Y = 63 };
Console.WriteLine(ob);
//ob.X = 70;
//ob.Y = 90;
}
}
}
Output

Fit and Finish Features
It is possible to omit the type when creating a new object and the type of object being created is already know. Therefore, we can use the new expression as given below.
using System;
namespace ConsoleApp39
{
class A
{
public int X { get; init; }
public int Y { get; init; }
public override string ToString()
{
return $"Sum ={X+Y}";
}
}
class Program
{
static void Main(string[] args)
{
A ob1 = new();
Console.WriteLine(ob1);
}
}
}
Module Initializers
Basically, ModuleInitializer is an attribute that we can use with a method. The module initializers are executed before any field is accessed or any other method is called. For example, look at the following code.
using System;
using System.Runtime.CompilerServices;
namespace ConsoleApp40
{
class Program
{
public static int x;
public static int y;
public static string AuthorName;
static void Main(string[] args)
{
Console.WriteLine($"Author Name: {AuthorName}");
Console.WriteLine($"Sum = {x+y}");
x = 80;y = 90;
Console.WriteLine($"Sum = {x + y}");
f();
Console.WriteLine($"Sum = {x + y}");
}
[ModuleInitializer]
public static void f()
{
x = 12; y = 16;
}
[ModuleInitializer]
public static void f1()
{
AuthorName = "Kavita";
}
}
}
Output

As shown above, we have two module initializers – f() and f1(). Evidently, we can have more than one module initializers. They will be executed first and only once implicitly and can assign values to static fields. After that, we can all them explicitly.
Another key point about object initializers is that they must be static and should have void return type. Also they should not accept any parameter and must be accessible using either public or internal access modifier.
Top-Level Programs
In fact, C# 9.0 offers great flexibility for writing programs quickly using Top-Level Programs. Therefore, it is not necessary to write the boilerplate code and you can just write any valid program statements as shown below.
using System;
using System.Collections;
Console.WriteLine("Welcome to C# 9.0");
ArrayList al = new();
al.Add("a");
al.Add("b");
al.Add(100);
al.Add(4.5);
Console.WriteLine("An Example of Array List...");
foreach (object i in al)
Console.Write(i + " ");
Output

Summary
C# 9.0 has added many new features. It is basically a part of .NET Framework 5.0. In general, records provide us many benefits that are not available in classes. Also, the init-only properties allow us to have readonly properties that we can use with object-initializer syntax. Moreover, C# 9.0 simplifies the code development by providing top-level statements. Finally, module initializers allow us to work on the static fields before any other code is executed.
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
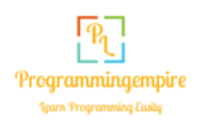